Scheduling algorithms are a crucial part of operating systems, tasked with efficiently managing the execution of processes. For students studying C++, understanding these algorithms can be both challenging and rewarding. This blog will delve into some common scheduling algorithms, their implementations in C++, and how getting C++ assignment help can streamline your learning process.
Understanding Scheduling Algorithms
Scheduling algorithms determine the order in which processes are executed in a system. They play a significant role in optimizing performance, reducing waiting times, and ensuring fairness. Here are a few widely-used scheduling algorithms:
First-Come, First-Served (FCFS)
Description: FCFS is one of the simplest scheduling algorithms. It processes tasks in the order they arrive. While easy to implement, it can lead to the "convoy effect," where short tasks wait behind long ones.
Implementation in C++: To implement FCFS, you would maintain a queue of processes and execute them in sequence. The C++ code involves creating a queue data structure and iterating through it to execute each process.
Shortest Job Next (SJN)
Description: SJN, also known as Shortest Job First (SJF), schedules processes with the shortest burst time first. This approach minimizes the average waiting time but requires knowing the execution time of each process in advance.
Implementation in C++: Implementing SJN involves sorting processes based on their burst time and then executing them in the sorted order. You can use arrays or priority queues in C++ to manage and sort these processes.
Round Robin (RR)
Description: RR is a preemptive scheduling algorithm where each process is assigned a fixed time slice or quantum. After the quantum expires, the process is moved to the back of the queue, and the next process is executed.
Implementation in C++: To implement RR in C++, you'll use a queue to hold processes and iterate through the queue, ensuring each process gets an equal time slice. The process will be put back in the queue if it isn't finished within its time slice.
Priority Scheduling
Description: This algorithm assigns a priority to each process and schedules the highest priority process first. If two processes have the same priority, FCFS is used to determine their order.
Implementation in C++: Implementing priority scheduling involves using a priority queue or heap data structure to manage processes based on their priority levels.
Why C++ Assignment Help Can Make a Difference
Implementing scheduling algorithms in C++ can be complex, particularly for students who are new to the language or the algorithms themselves. Here’s how C++ assignment help can assist you:
Understanding Core Concepts:
Clarification: Assignment help services can provide detailed explanations of scheduling algorithms and their implementation in C++, helping you grasp the fundamental concepts.
Examples: You can receive well-commented code examples demonstrating how to implement different algorithms, which aids in understanding and learning.
Efficient Coding:
Optimized Code: Professional assistance ensures that your code is efficient and adheres to best practices, avoiding common pitfalls such as inefficient data structures or unnecessary computations.
Debugging: Help services can assist in identifying and fixing bugs in your implementation, ensuring your scheduling algorithms work as intended.
Time Management:
Focused Learning: By outsourcing complex assignments, you can focus on learning and understanding the theoretical aspects of scheduling algorithms without getting bogged down by implementation issues.
Deadlines: Meeting assignment deadlines becomes more manageable with expert help, allowing you to submit high-quality work on time.
Personalized Assistance:
Tailored Solutions: Assignment help services offer solutions tailored to your specific requirements, whether you need help with understanding a particular algorithm or implementing it in C++.
Feedback: You can receive constructive feedback and tips for improvement, enhancing your overall learning experience.
Conclusion
Scheduling algorithms are a fundamental aspect of operating systems and an essential topic for C++ programming students. Mastering these algorithms can greatly enhance your understanding of process management and optimization. If you find yourself struggling with implementing these algorithms or need extra help with your C++ assignments, consider seeking C++ assignment help. Professional assistance can make a significant difference in your learning journey, helping you achieve better results and deeper insights into the world of scheduling algorithms.
Reference: https://www.programminghomewor....khelp.com/blog/sched
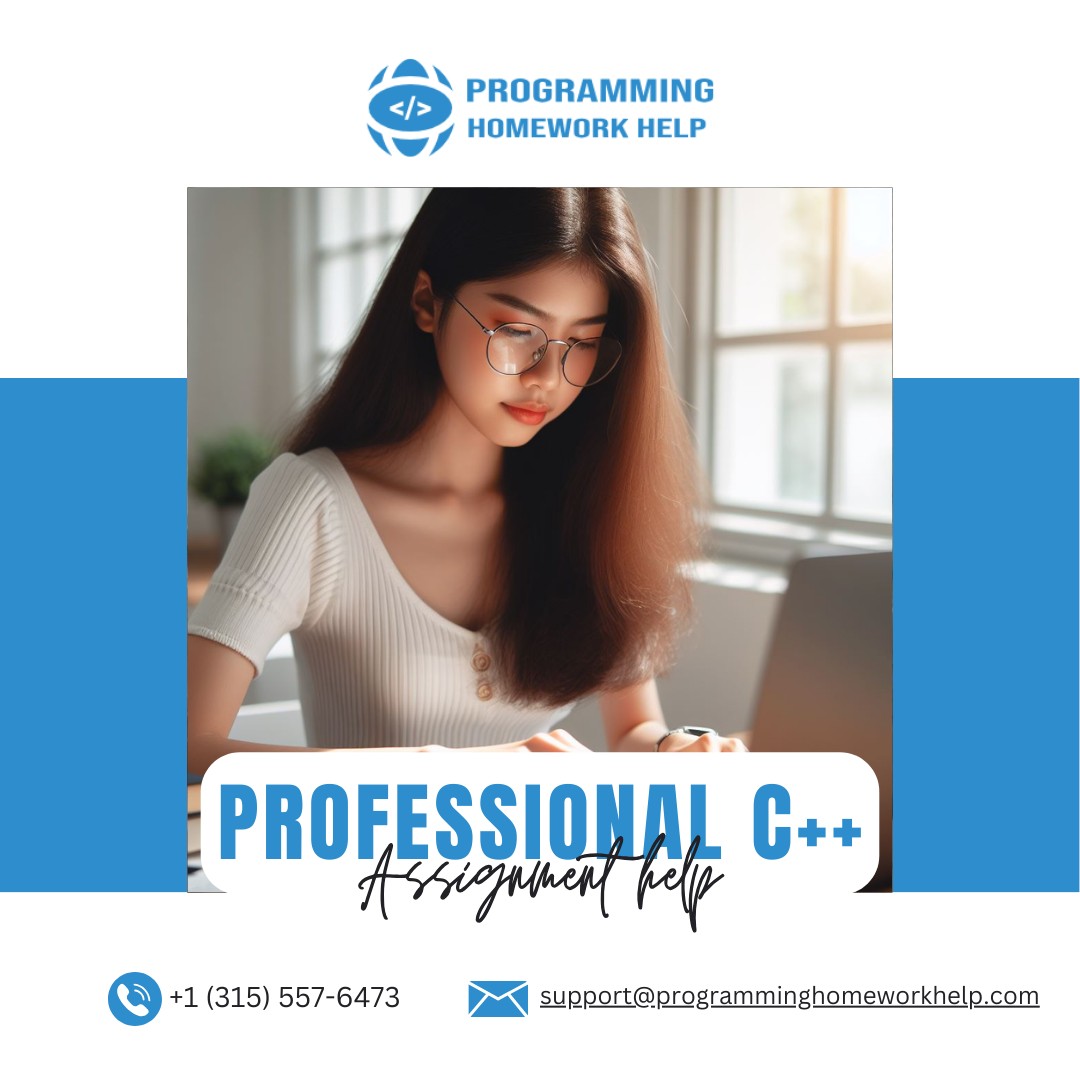